Server Action Scripts
Service Actions are ServiceNow scripts that a flow can execute to enable Sofi to interact with ServiceNow. Typical interactions include:
- Table data lookup
- Choice list (reference lookup)
- Create / update record
Service Actions can located on the ServiceNow Sofi application:
Sofi > Configuration > Server Actions
Server Actions have the following configuration parameters.
Field | Description |
---|---|
Name | Name of the Server Action |
Type | Choice List Lookup Log Ticket Legacy * Custom |
Description | Description of the server action. |
Script | Service action script |
Choice List Server Actions
Choice list lookups are two phase:
- 'list' phase: Creates a ServiceNow query and returns the data to be displayed in the Choice List
- 'action' phase: Receives the data and takes an action.
Example Choice List Server Action
// Lookups are two phase:
// 'list' phase: Creates a ServiceNow query and returns the data to be displayed in the Choice List
// 'action' phase: Receives the data and takes an action.
switch (action.phase) {
case "list":
// Get the list of users matching the search
var dataColumns = ["name", "email", "phone", "sys_id"];
// Get the set of users to return, and include the name, email, phone and sys_id
// in the results
answer = {
records: SoapboxAIUtil.grToArray(
getUserQuery(action.search_text),
["name", "email", "phone", "sys_id"]
),
// Setup the display columns on the UI.
columns: [
{ name: "name", label: "Name", display: true, returnValue: true },
{ name: "email", label: "Email", display: true },
{ name: "phone", label: "Phone", display: true }
]
};
break;
case "action":
// Place the result in the variable passed in, or provide a default...
var variableName = SoapboxAIUtil.isBlankOrEmpty(action.variable_name) ? "selected_user" : action.variable_name;
// Save the data to the context for later use
context[variableName] = action.data;
answer = "ok";
break;
}
function getUserQuery(searchString) {
var rec = new GlideRecord("sys_user");
rec.addEncodedQuery(
'nameLIKE' + action.search_text +
'^ORphoneLIKE'+action.search_text +
'^ORemailLIKE'+action.search_text
);
rec.orderBy("name");
rec.setLimit(15);
return rec;
}
Log Ticket Server Actions
Log ticket actions are required to process a log ticket request, log the appropriate ticket, and return a defined set of information which can be used to provide the end user with the updated ticket information.
Default Log Ticket Server Action
// Use the built-in DialogManager Helper to create the ticket and populate it.
var rec = SoapboxAIDialog.createRecordForLogTicketAction(action);
// Preform any required modifications to the ticket here...
// Update and return the record details.
answer = SoapboxAIDialog.updateRecordAndReturnValues(rec, action);
Lookup Server Actions
Lookup server actions are called from Lookup elements. They return one or more responses that correspond to branches on the Lookup element.
Example Lookup Server Action
In the following example the lookup server action checks the value of the locked_out and active fields of the user Abel.tuter.
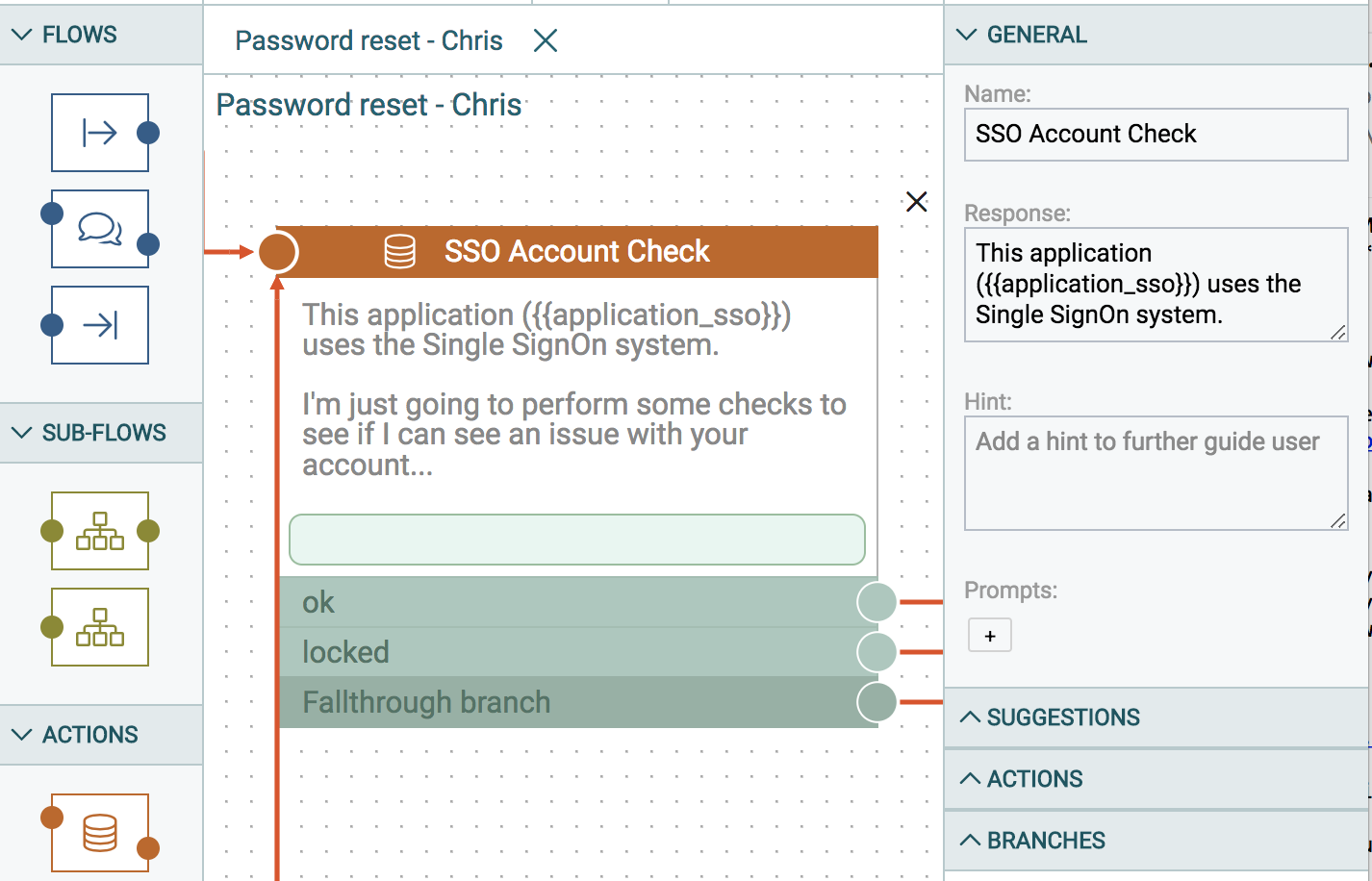
// Uses Abel Tutors account as the baseline for the test
var userRec = SoapboxAIUtil.lookupUniqueRec("sys_user", ["user_name", "abel.tuter"]);
if (userRec.locked_out == true) {
answer = "locked";
} else if (userRec.active == false) {
answer = "disabled";
} else if (userRec.password_needs_reset == true) {
answer = "reset";
} else {
answer = "ok";
}
Updated about 7 years ago